Selenium Webdriver Tutorial with Examples
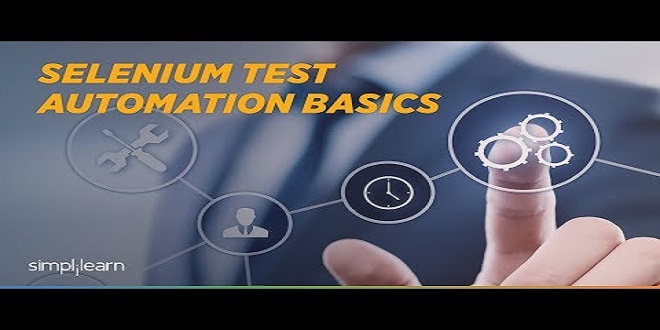
The web application landscape has experienced rapid expansion and evolution in recent years, necessitating robust and efficient testing frameworks to ensure product quality. One such pivotal technology that has transformed the realm of web application testing is Selenium WebDriver.
Originally a project born out of ThoughtWorks by Jason Huggins in 2004, Selenium has become the de facto standard for web application testing. Selenium WebDriver, the successor to Selenium RC, was introduced to overcome limitations in the original Selenium tool, offering a more robust and object-oriented API, and improved support for modern advanced web-app testing problems.
The importance of Selenium WebDriver in the field of software testing is difficult to overstate. Its ability to automate browser activities and simulate user interactions on web applications makes it an invaluable tool for functional and regression testing. Its compatibility with various programming languages, browsers, and operating systems further enhances its appeal, making it an ideal choice for testing in diverse environments.
In this blog post, we will explore the world of Selenium WebDriver. We aim to cover the fundamentals, including its installation and setup, creating your first Selenium WebDriver script, working with various web elements, and managing waits. We will also delve into handling pop-ups and alerts, implementing cross-browser testing, and sharing some of the best practices for using this powerful testing tool. Join us as we embark on this fascinating journey to master Selenium WebDriver.
1. Pre-requisites
Before you dive into using Selenium WebDriver, it’s important to have a basic understanding of certain technologies. Knowledge of HTML, JavaScript, and CSS is crucial as they form the basis of web development. Understanding these languages will aid you in writing test scripts, identifying web elements, and debugging your code.
In terms of software, you will need to have the Java Development Kit (JDK) installed on your machine, as we’ll be using Java for our test scripts in this tutorial. An integrated development environment (IDE) such as Eclipse is also essential for writing and executing your scripts. Of course, Selenium WebDriver is necessary as the primary tool for automating the browser. Lastly, depending on the browser in which you wish to execute your tests (such as Firefox, Chrome, etc.), you will need to install the corresponding browser drivers.
2. Installing and Setting up Selenium WebDriver
Getting started with Selenium WebDriver involves a few installation and setup steps:
- Install JDK and Eclipse: Firstly, you need to install JDK (Java Development Kit) as it is a pre-requisite to run Selenium WebDriver. After that, install Eclipse IDE, which will be used for writing our Selenium Scripts.
- Install Selenium WebDriver and Setup Browser Drivers: You can download Selenium WebDriver from the official Selenium website. It comes as a .jar file which you will need to add to your Java project in Eclipse. For browser drivers, you need to download the ones corresponding to the browsers you wish to use (like geckodriver for Firefox, chromedriver for Chrome, etc.) from their official websites. After downloading, you need to set the path of these browser drivers in your Java code using System.setProperty() method.
- Ensure Correct Installation and Setup: To ensure that you have set up everything correctly, write a simple script that opens a web page on your browser. If the page loads successfully, it means that the Selenium WebDriver has been correctly installed and configured. If not, check your setup and installation steps. Remember, the success of your future automation scripts greatly depends on this initial setup, so take your time to get it right.
3. Understanding WebDriver and Web Elements
Understanding the architecture of Selenium WebDriver and the concept of Web Elements is essential for effective automation testing. Selenium WebDriver’s architecture consists of four key components: language bindings, JSON Wire Protocol over HTTP, browser drivers, and browsers. Language bindings allow you to write your scripts in various programming languages. JSON Wire Protocol acts as a medium of communication between your scripts and browser drivers. The browser drivers interpret the scripts into actions for the respective browsers.
Web Elements, on the other hand, are the building blocks of any web page. These can be buttons, text boxes, images, links, etc. In WebDriver, we interact with these Web Elements to perform actions like entering text, clicking buttons, selecting from a dropdown, among others.
Selenium WebDriver offers various methods to interact with these elements, such as findElement(), click(), sendKeys(), etc. Similarly, Web Elements also have methods like getText(), getAttribute(), and more. Familiarizing yourself with these methods is crucial for efficient automation scripting.
4. Your First Selenium WebDriver Script
Creating your first Selenium WebDriver script is an exciting milestone. Let’s create a simple script that opens a webpage and interacts with it:
- Step-by-step Guide
- Create a new project in Eclipse.
- Download and add the Selenium WebDriver .jar files to your project.
- Set the browser driver’s path using System.setProperty().
- Instantiate a new WebDriver object.
- Open a webpage using the get() method.
- Interact with a Web Element using the appropriate WebDriver method.
- Close the browser using the quit() method.
- Code Snippet
System.setProperty(“webdriver.chrome.driver”, “path_to_chromedriver”);
WebDriver driver = new ChromeDriver();
driver.get(“https://www.example.com”);
WebElement element = driver.findElement(By.name(“q”));
element.sendKeys(“Selenium WebDriver”);
element.submit();
driver.quit();
In this script, we are opening Google’s homepage, finding the search box, entering “Selenium WebDriver”, submitting the query, and finally closing the browser.
- Running and Verifying the Script
To run the script, simply click on the Run button in Eclipse. Verify that the script executes as expected by watching the automated actions in the browser and checking that no errors are displayed in the Eclipse console. If the script executes successfully, congratulations on creating your first Selenium WebDriver script!
5. Working with Different Web Elements
Web elements are the various components of a web page, and interacting with these elements forms the crux of Selenium WebDriver scripting. Below are some common web elements and examples of how to work with them:
- Working with Different Web Elements
- Text Boxes: To interact with a text box, you can use the sendKeys() method. This allows you to type a string of text into the text box as if you were a user typing.
- Buttons: Buttons can be clicked using the click() method. This could be a submit button, a next button, or any other type of command button.
- Check Boxes and Radio Buttons: These can also be selected using the click() method. However, it’s often good practice to first check if they are already selected using isSelected() before attempting to click.
- Drop-down Menus: Selenium WebDriver provides the Select class to interact with drop-down menus. You can select options by visible text, by value, or by index.
- Code Examples
// Text Box
WebElement textBox = driver.findElement(By.id(“textbox-id”));
textBox.sendKeys(“Example text”);
// Button
WebElement button = driver.findElement(By.id(“button-id”));
button.click();
// Check Box
WebElement checkBox = driver.findElement(By.id(“checkbox-id”));
if (!checkBox.isSelected()) {
checkBox.click();
// Drop-down Menu
Select dropdown = new Select(driver.findElement(By.id(“dropdown-id”)));
dropdown.selectByVisibleText(“Option”);
- Tips and Common Issues
Always ensure that the element is interactable (visible and enabled) before attempting to interact with it. Also, it’s crucial to correctly identify the elements using the appropriate locator strategy (ID, Name, Class Name, etc.). Incorrect or weak locators are a common source of issues in Selenium scripts.
6. Implicit and Explicit Waits in WebDriver
- Importance of Using Waits
Web applications often contain elements that may not be immediately available for interaction. This could be due to animations, AJAX calls, or simply the time it takes for the server to respond. To handle such scenarios, Selenium provides waits.
- Differences Between Implicit and Explicit Waits
Implicit wait tells WebDriver to poll the DOM for a certain amount of time when trying to find an element or elements if they are not immediately available. The default setting is 0.
Explicit wait is used to halt the execution until a specific condition is met or the maximum time has elapsed. Unlike implicit waits, explicit waits are applied only for specified elements.
- Code Examples
// Implicit Wait
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Explicit Wait
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“element-id”)));
In these examples, WebDriver will wait up to 10 seconds for an element to appear before throwing an exception. The exact time waited will depend on when the element becomes available.
7. Handling Pop-ups, Alerts, and Multiple Windows
- Handling Pop-ups and Alerts with WebDriver
Pop-ups and alerts are common in web applications. Selenium WebDriver provides the Alert interface to handle these. Alert offers methods such as accept(), dismiss(), and getText() to interact with alerts.
- Switching Between Multiple Windows
In scenarios where interaction with multiple browser windows is required, WebDriver’s getWindowHandles() and switchTo() methods come to the rescue. getWindowHandles() returns a set of window handles which can be used to switch between windows.
- Handling Common Challenges and Best Practices
One common challenge when dealing with pop-ups, alerts, or multiple windows is ensuring the correct window or alert is being interacted with. Always validate that you’re in the right context before interacting with elements.
8. Cross Browser Testing using Selenium WebDriver
- Need for Cross-Browser Testing
Cross-browser testing is crucial to ensure a consistent user experience across different browsers. Due to differences in the way browsers render HTML and CSS, a web application might behave or display differently across browsers.
- Code Examples of Cross-Browser Tests
With Selenium WebDriver, you can set up your tests to run on multiple browsers. Below is an example of a test set to run on Chrome and Firefox.
public void runTestOnMultipleBrowsers() {
WebDriver driver;
String[] browsers = {“chrome”, “firefox”};
for (String browser : browsers) {
if(browser.equals(“chrome”)) {
System.setProperty(“webdriver.chrome.driver”, “path_to_chromedriver”);
driver = new ChromeDriver();
} else if(browser.equals(“firefox”)) {
System.setProperty(“webdriver.gecko.driver”, “path_to_geckodriver”);
driver = new FirefoxDriver();
driver.get(“https://www.example.com”);
// rest of the test steps
driver.quit();
- Tips for Effective Cross-Browser Testing
Maintaining separate profiles for different browsers can help manage browser-specific settings. Additionally, leveraging unified digital experience testing platforms like LambdaTest can improve testing efficiency and coverage with a fraction of the cost of inhouse device lab or private grid.
9. Best Practices and Tips for Using Selenium WebDriver
- Highlighting Key Best Practices
- Use Appropriate Locators: Prefer ID and Name locators as they are more efficient and reliable. XPath and CSS Selector are powerful but can be complex and brittle.
- Keep Tests Independent: Each test should be able to run independently without dependencies on other tests. This improves the readability and reliability of your tests.
- Use Page Object Model: This design pattern improves maintainability by separating the page structure from the test scripts.
- Tips for Efficient Scripting and Troubleshooting
- Handle Delays: Use explicit waits to handle dynamic content or page loading times.
- Error Handling: Incorporate proper error handling in your scripts. This helps in better debugging when a test fails.
Conclusion
In this blog, we have explored the multifaceted tool that is Selenium WebDriver. From understanding its architecture and working with web elements, handling pop-ups, alerts, and multiple windows, to performing cross-browser testing, we’ve covered a lot of ground. Remember, the key to mastering Selenium WebDriver lies in hands-on practice. So, don’t hesitate to get your hands dirty, write code, learn from your mistakes, and keep iterating. Happy Testing!